Laravel 9 Accessor and Mutator with an example
Laravel Accessors and mutators allow you to transform Eloquent attribute values when you retrieve or set them on model instances.
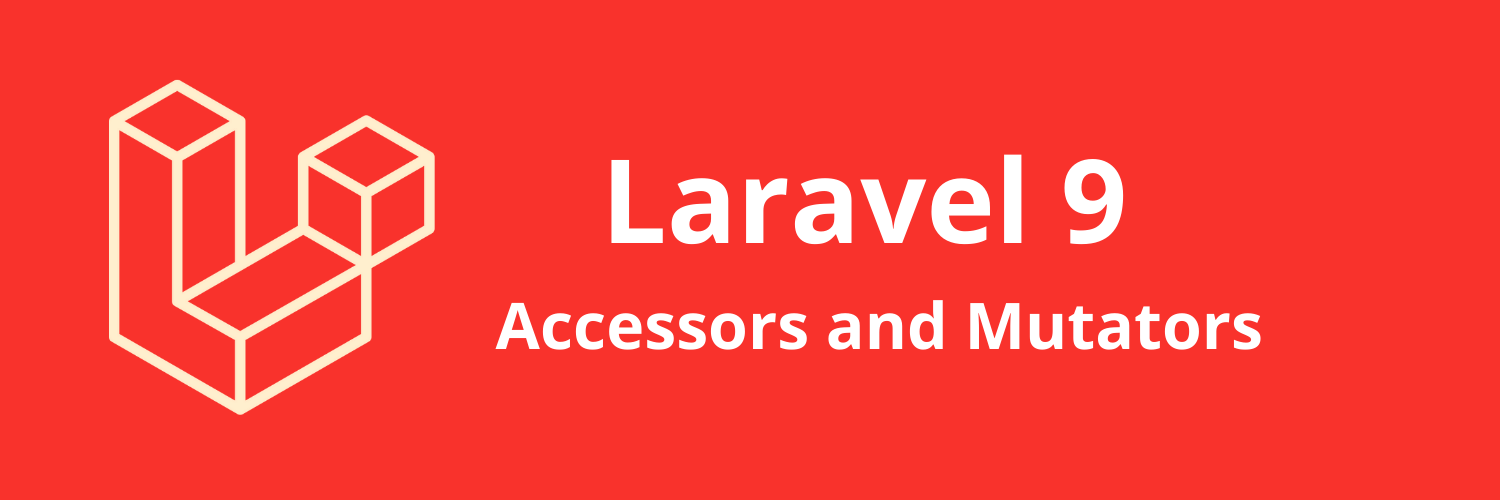
An accessor is used to alter the Eloquent attribute value when it is accessed and the mutator is used to alter the value before it is set to the database.
Let’s dive into the example 👇
I am assuming that you have a basic understanding of Laravel Eloquent.
We will consider the example of `Book`.
let’s create a migration for the “books” table using below command.
php artisan make:migration create_books_table
Now, our migration has been created. open the migration file and replace below code with the ‘up’ method.
public function up()
{
Schema::create('books', function (Blueprint $table) {
$table->id();
$table->string("title");
$table->longText("summary");
$table->timestamps();
});
}
After that, run below command to execute the migration.
php artisan migrate
Next, we need to create the Eloquent model using the below command.
php artisan make:model Book
Now we need to define the accessor and mutator in Modal. so replace the below code with the Book.php.
<?php
namespace App\Models;
use Illuminate\Database\Eloquent\Factories\HasFactory;
use Illuminate\Database\Eloquent\Model;
use Illuminate\Database\Eloquent\Casts\Attribute;
class Book extends Model
{
use HasFactory;
protected function title(): Attribute
{
return Attribute::make(
get: fn($value) => ucfirst($value), Â // accessor
set: fn ($value) => strtolower($value), Â // mutator
);
}
}
In Book.php, we defined the protected method title() for the title column. The column name must be same as the method name. If the column has underscore in its name then method should be in the camel case e.g. if the column name is first_name then method name should be firstName().
The accessor and mutator will be automatically called when attemping to retrieve and insert the value to the database. so when we access the value then get argument will be called automatically and set argument will be called when value will be set to the model.
Now we need to create the controller using the below command.
php artisan make:controller BookController
Replace the below code with the BookController.php
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use App\Models\Book;
class BookController extends Controller
{
  public function index(){
    return Book::all();
  }
public function store(){
$book = new Book;
$book->title = "Book title";
$book->summary = "This is book summary";
$book->save();
}
}
we are creating a new book entry in the store method. It will set the title column value in lower case because we have used strtolower
in mutator method.
In the index method, we are using Book
modal to load the data from the database. The first character of the title will be in uppercase because we have used ucfirst
method in the accessor method.
so at last, Â we need to call controller methods from the routing. so replace the below code with the web.php
<?php
use Illuminate\Support\Facades\Route;
use App\Http\Controllers\BookController;
Route::get('/', [BookController::class, "index"]);
Route::get("book", [BookController::class, "create"]);
Route::post("book", [BookController::class, "store"]);
Thank you for reading the article.